mirror of
https://github.com/chibicitiberiu/rainmeter-studio.git
synced 2024-02-24 04:33:31 +00:00
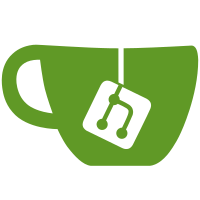
[Measure] Disabled [Meter] Hidden X Y In case of "Hidden": - Hidden not added or Hidden=0/1 (=fixed value specified) and DynamicVariables=1 The specified value is handled as the starting value. After that the value is not re-read on every update. !RainmeterShowMeter etc. are enabled. - Hidden=#VAR# or Hidden=[Measure] and DynamicVariables=1 The value is re-read on every update. !RainmeterShowMeter etc. are disabled by re-reading value.
105 lines
4.0 KiB
C++
105 lines
4.0 KiB
C++
/*
|
|
Copyright (C) 2004 Kimmo Pekkola
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public License
|
|
as published by the Free Software Foundation; either version 2
|
|
of the License, or (at your option) any later version.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; if not, write to the Free Software
|
|
Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
|
|
*/
|
|
|
|
#ifndef __CONFIGPARSER_H__
|
|
#define __CONFIGPARSER_H__
|
|
|
|
#include <windows.h>
|
|
#include <map>
|
|
#include <string>
|
|
#include <vector>
|
|
#include <hash_map>
|
|
#include <gdiplus.h>
|
|
#include "ccalc-0.5.1/mparser.h"
|
|
|
|
class CRainmeter;
|
|
class CMeterWindow;
|
|
class CMeasure;
|
|
|
|
class CConfigParser
|
|
{
|
|
public:
|
|
CConfigParser();
|
|
~CConfigParser();
|
|
|
|
void Initialize(LPCTSTR filename, CRainmeter* pRainmeter, CMeterWindow* meterWindow = NULL);
|
|
void AddMeasure(CMeasure* pMeasure);
|
|
void SetVariable(const std::wstring& strVariable, const std::wstring& strValue);
|
|
void SetStyleTemplate(const std::wstring& strStyle) { m_StyleTemplate = strStyle; }
|
|
void ResetStyleTemplate() { m_StyleTemplate.clear(); }
|
|
|
|
void ResetVariables(CRainmeter* pRainmeter, CMeterWindow* meterWindow = NULL);
|
|
|
|
const std::wstring& ReadString(LPCTSTR section, LPCTSTR key, LPCTSTR defValue, bool bReplaceMeasures = true, bool* bReplaced = NULL);
|
|
double ReadFloat(LPCTSTR section, LPCTSTR key, double defValue);
|
|
double ReadFormula(LPCTSTR section, LPCTSTR key, double defValue);
|
|
int ReadInt(LPCTSTR section, LPCTSTR key, int defValue);
|
|
Gdiplus::Color ReadColor(LPCTSTR section, LPCTSTR key, const Gdiplus::Color& defValue);
|
|
std::vector<Gdiplus::REAL> ReadFloats(LPCTSTR section, LPCTSTR key);
|
|
|
|
std::wstring& GetFilename() { return m_Filename; }
|
|
const std::vector<std::wstring>& GetSections();
|
|
|
|
// Returns an int if the formula was read successfully, -1 for failure.
|
|
int ReadFormula(const std::wstring& result, double* number);
|
|
|
|
static std::vector<std::wstring> Tokenize(const std::wstring& str, const std::wstring delimiters);
|
|
static double ParseDouble(const std::wstring& string, double defValue, bool rejectExp = false);
|
|
static Gdiplus::Color ParseColor(LPCTSTR string);
|
|
|
|
static void ClearMultiMonitorVariables() { c_MonitorVariables.clear(); }
|
|
static void UpdateWorkareaVariables() { SetMultiMonitorVariables(false); }
|
|
|
|
private:
|
|
void SetDefaultVariables(CRainmeter* pRainmeter, CMeterWindow* meterWindow);
|
|
void ReadVariables();
|
|
bool ReplaceVariables(std::wstring& result);
|
|
bool ReplaceMeasures(std::wstring& result);
|
|
|
|
void ReadIniFile(const std::wstring& strFileName, int depth = 0);
|
|
void SetValue(const std::wstring& strSection, const std::wstring& strKey, const std::wstring& strValue);
|
|
const std::wstring& GetValue(const std::wstring& strSection, const std::wstring& strKey, const std::wstring& strDefault);
|
|
std::vector<std::wstring> GetKeys(const std::wstring& strSection);
|
|
|
|
void SetAutoSelectedMonitorVariables(CMeterWindow* meterWindow);
|
|
|
|
void GetIniFileMappingList();
|
|
std::wstring GetAlternateFileName(const std::wstring& iniFile);
|
|
|
|
static void SetMultiMonitorVariables(bool reset);
|
|
static void SetMonitorVariable(const std::wstring& strVariable, const std::wstring& strValue);
|
|
|
|
std::map<std::wstring, std::wstring> m_Variables;
|
|
std::wstring m_Filename;
|
|
|
|
hqMathParser* m_Parser;
|
|
std::map<std::wstring, CMeasure*> m_Measures;
|
|
|
|
std::wstring m_StyleTemplate;
|
|
|
|
std::vector<std::wstring> m_Sections; // The sections must be an ordered array
|
|
stdext::hash_map<std::wstring, std::vector<std::wstring> > m_Keys;
|
|
stdext::hash_map<std::wstring, std::wstring> m_Values;
|
|
|
|
std::vector<std::wstring> m_IniFileMappings;
|
|
|
|
static std::map<std::wstring, std::wstring> c_MonitorVariables;
|
|
};
|
|
|
|
#endif
|