mirror of
https://github.com/chibicitiberiu/rainmeter-studio.git
synced 2024-02-24 04:33:31 +00:00
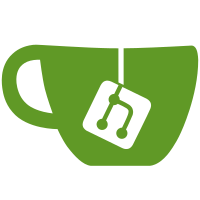
The height is now based on a formula that should be exactly equal to that provided by GDI+. The padding should now be equal on the left and right sides now. There may be some discrepancies in overall width, but it should always be reasonably to close to GDI+. In addition, this makes D2D behavior match GDI+ when a newline character is the last character of the text.
75 lines
2.4 KiB
C++
75 lines
2.4 KiB
C++
/*
|
|
Copyright (C) 2013 Birunthan Mohanathas
|
|
|
|
This program is free software; you can redistribute it and/or
|
|
modify it under the terms of the GNU General Public License
|
|
as published by the Free Software Foundation; either version 2
|
|
of the License, or (at your option) any later version.
|
|
|
|
This program is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program; if not, write to the Free Software
|
|
Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
*/
|
|
|
|
#ifndef RM_GFX_TEXTFORMATD2D_H_
|
|
#define RM_GFX_TEXTFORMATD2D_H_
|
|
|
|
#include "TextFormat.h"
|
|
#include <string>
|
|
#include <dwrite_1.h>
|
|
#include <wrl/client.h>
|
|
|
|
namespace Gfx {
|
|
|
|
// Provides a Direct2D/DirectWrite implementation of TextFormat for use with CanvasD2D.
|
|
class TextFormatD2D : public TextFormat
|
|
{
|
|
public:
|
|
TextFormatD2D();
|
|
virtual ~TextFormatD2D();
|
|
|
|
virtual bool IsInitialized() const override { return m_TextFormat != nullptr; }
|
|
|
|
virtual void SetProperties(
|
|
const WCHAR* fontFamily, int size, bool bold, bool italic,
|
|
const FontCollection* fontCollection) override;
|
|
|
|
virtual void SetTrimming(bool trim) override;
|
|
|
|
virtual void SetHorizontalAlignment(HorizontalAlignment alignment) override;
|
|
virtual void SetVerticalAlignment(VerticalAlignment alignment) override;
|
|
|
|
private:
|
|
friend class CanvasD2D;
|
|
|
|
TextFormatD2D(const TextFormatD2D& other) {}
|
|
|
|
void Dispose();
|
|
|
|
// Creates a new DirectWrite text layout if |str| has changed since last call. Since creating
|
|
// the layout is costly, it is more efficient to keep reusing the text layout until the text
|
|
// changes.
|
|
void CreateLayout(const WCHAR* str, UINT strLen, float maxW, float maxH, bool gdiEmulation);
|
|
|
|
DWRITE_TEXT_METRICS GetMetrics(
|
|
const WCHAR* str, UINT strLen, bool gdiEmulation, float maxWidth = 10000.0f);
|
|
|
|
Microsoft::WRL::ComPtr<IDWriteTextFormat> m_TextFormat;
|
|
Microsoft::WRL::ComPtr<IDWriteTextLayout> m_TextLayout;
|
|
Microsoft::WRL::ComPtr<IDWriteInlineObject> m_InlineEllipsis;
|
|
|
|
std::wstring m_LastString;
|
|
|
|
// Used to emulate GDI+ behaviour.
|
|
float m_ExtraHeight;
|
|
float m_LineGap;
|
|
};
|
|
|
|
} // namespace Gfx
|
|
|
|
#endif |